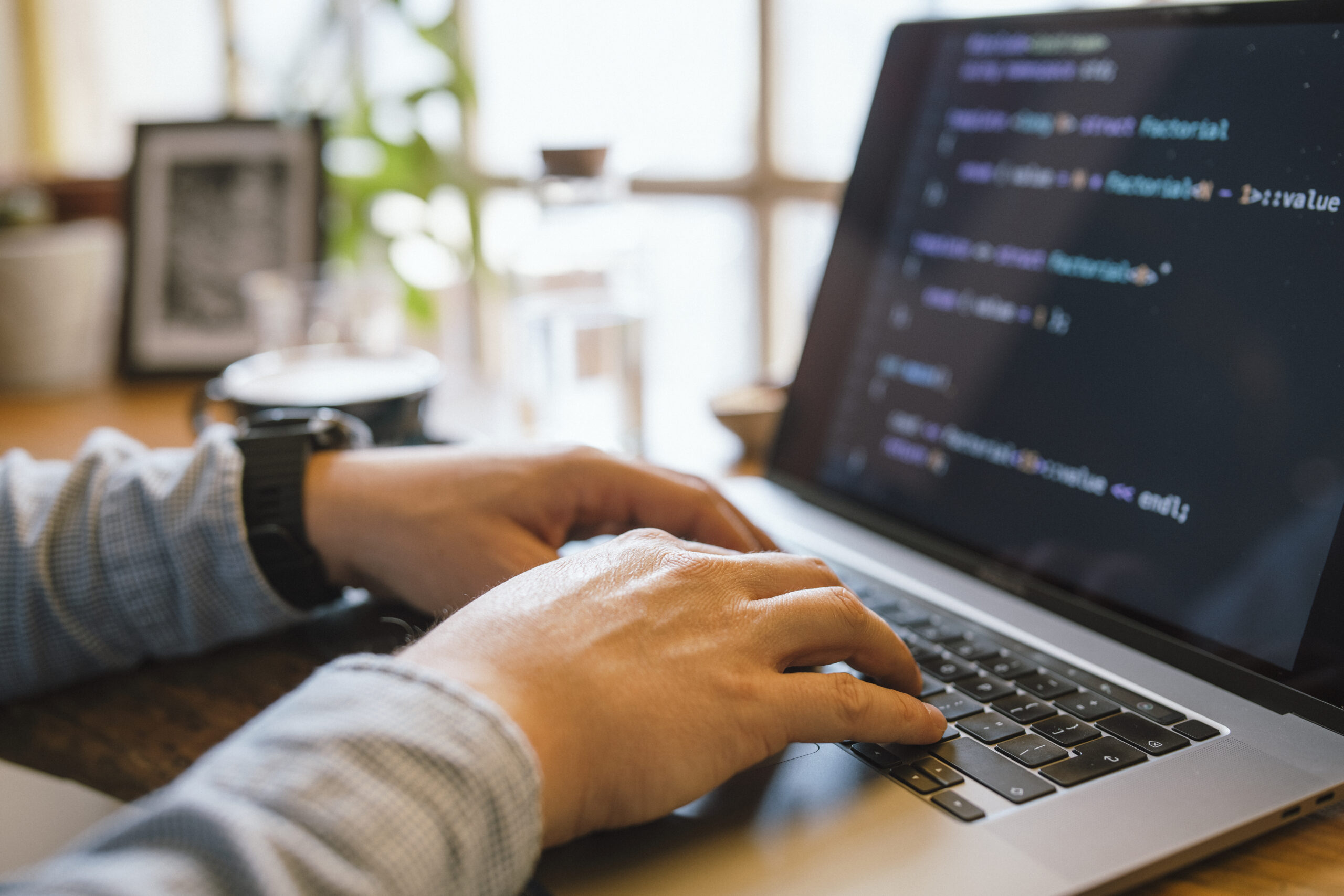
Debugging is Probably the most crucial — still normally ignored — expertise in the developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and Finding out to Assume methodically to unravel challenges competently. Whether you are a newbie or even a seasoned developer, sharpening your debugging expertise can preserve hrs of aggravation and drastically transform your productiveness. Allow me to share many approaches to aid developers level up their debugging sport by me, Gustavo Woltmann.
Master Your Applications
Among the list of fastest techniques developers can elevate their debugging expertise is by mastering the resources they use every day. Though producing code is one particular Portion of growth, realizing how you can connect with it proficiently for the duration of execution is equally essential. Fashionable progress environments arrive Geared up with strong debugging capabilities — but lots of builders only scratch the surface area of what these tools can perform.
Consider, for example, an Integrated Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, phase by means of code line by line, and perhaps modify code over the fly. When utilised appropriately, they Permit you to observe just how your code behaves in the course of execution, which is a must have for tracking down elusive bugs.
Browser developer applications, for instance Chrome DevTools, are indispensable for front-finish builders. They enable you to inspect the DOM, keep track of community requests, look at authentic-time functionality metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can turn annoying UI challenges into workable tasks.
For backend or program-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB offer deep Command more than jogging procedures and memory management. Understanding these instruments may have a steeper Mastering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, become cozy with Model Command programs like Git to be familiar with code history, discover the exact second bugs have been released, and isolate problematic changes.
In the end, mastering your resources signifies heading outside of default options and shortcuts — it’s about developing an intimate knowledge of your improvement surroundings to ensure when troubles occur, you’re not shed in the dark. The better you know your tools, the greater time you could expend solving the actual problem rather than fumbling through the procedure.
Reproduce the situation
Among the most critical — and infrequently missed — techniques in productive debugging is reproducing the challenge. Just before jumping to the code or producing guesses, developers have to have to produce a regular surroundings or scenario in which the bug reliably appears. Without the need of reproducibility, repairing a bug turns into a game of prospect, typically bringing about wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Request questions like: What steps led to The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or mistake messages? The more depth you've, the a lot easier it gets to isolate the precise problems beneath which the bug takes place.
As soon as you’ve gathered adequate information, try and recreate the issue in your neighborhood setting. This may suggest inputting the same info, simulating identical user interactions, or mimicking process states. If The problem seems intermittently, contemplate producing automated exams that replicate the sting cases or point out transitions involved. These assessments don't just assist expose the situation but also avoid regressions in the future.
Often, The difficulty might be setting-unique — it might take place only on selected working programs, browsers, or less than particular configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these bugs.
Reproducing the problem isn’t just a stage — it’s a frame of mind. It involves tolerance, observation, along with a methodical technique. But as soon as you can continually recreate the bug, you might be already midway to correcting it. Using a reproducible situation, You need to use your debugging instruments additional proficiently, exam opportunity fixes properly, and communicate much more clearly together with your group or customers. It turns an abstract criticism right into a concrete obstacle — Which’s wherever builders thrive.
Go through and Realize the Error Messages
Mistake messages are sometimes the most useful clues a developer has when anything goes Improper. As opposed to seeing them as frustrating interruptions, builders really should master to take care of error messages as direct communications within the process. They typically let you know what exactly occurred, where it transpired, and often even why it happened — if you know the way to interpret them.
Start off by reading through the concept carefully As well as in total. Many builders, particularly when less than time force, glance at the very first line and straight away start off creating assumptions. But further inside the mistake stack or logs may possibly lie the true root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and understand them initially.
Break the mistake down into areas. Can it be a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line number? What module or functionality induced it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable designs, and Mastering to recognize these can dramatically increase your debugging approach.
Some faults are vague or generic, and in All those cases, it’s vital to look at the context wherein the mistake happened. Check connected log entries, enter values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings both. These frequently precede more substantial challenges and supply hints about possible bugs.
In the end, error messages are certainly not your enemies—they’re your guides. Discovering to interpret them appropriately turns chaos into clarity, serving to you pinpoint challenges faster, decrease debugging time, and become a much more productive and self-confident developer.
Use Logging Correctly
Logging is one of the most potent resources within a developer’s debugging toolkit. When employed properly, it offers true-time insights into how an application behaves, supporting you have an understanding of what’s going on underneath the hood without having to pause execution or move from the code line by line.
A fantastic logging tactic commences with recognizing what to log and at what amount. Popular logging degrees include things like DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for in-depth diagnostic info throughout improvement, INFO for typical gatherings (like prosperous start off-ups), WARN for potential concerns that don’t break the applying, ERROR for precise challenges, and Deadly when the system can’t continue on.
Avoid flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure important messages and decelerate your program. Concentrate on vital gatherings, condition adjustments, enter/output values, and significant choice details within your code.
Structure your log messages Plainly and regularly. Involve context, which include timestamps, request IDs, and performance names, so it’s easier to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs Allow you to keep track of how variables evolve, what problems are met, and what branches of logic are executed—all with no halting the program. They’re Specially valuable in creation environments where stepping by way of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a nicely-assumed-out logging method, you may lessen the time it will take to identify challenges, acquire deeper visibility into your apps, and Increase the Over-all maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not merely a technical activity—it is a method of investigation. To effectively establish and fix bugs, developers need to technique the procedure similar to a detective resolving a mystery. This state of mind aids stop working complicated concerns into manageable areas and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Consider the indicators of the situation: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, collect just as much relevant details as it is possible to with no jumping to conclusions. Use logs, examination circumstances, and consumer reviews to piece with each other a clear photograph of what’s happening.
Next, form hypotheses. Ask yourself: What can be producing this actions? Have any improvements not long ago been manufactured to your codebase? Has this challenge transpired just before under similar instances? The purpose is always to narrow down possibilities and detect potential culprits.
Then, take a look at your theories systematically. Try and recreate the trouble in a managed setting. In the event you suspect a selected purpose or element, isolate it and validate if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the results guide you closer to the reality.
Pay out shut notice to smaller particulars. Bugs normally conceal inside the minimum expected destinations—like a lacking semicolon, an off-by-one particular error, or a race issue. Be thorough and client, resisting the urge to patch the issue without the need of completely being familiar with it. Short term fixes may perhaps conceal the real dilemma, only for it to resurface later on.
Last of all, preserve notes on Anything you experimented with and acquired. Just as detectives log their investigations, documenting your debugging course of action can conserve time for future concerns and assistance Other people fully grasp your reasoning.
By thinking just like a detective, builders can sharpen their analytical competencies, strategy challenges methodically, and become more effective at uncovering concealed issues in intricate units.
Write Exams
Producing checks is among the most effective methods to increase your debugging abilities and All round progress performance. Checks not only assist catch bugs early but in addition serve as a safety Internet that provides you self esteem when earning modifications for your codebase. A effectively-examined application is simpler to debug since it lets you pinpoint just wherever and when a challenge occurs.
Start with device checks, which deal with individual capabilities or modules. These compact, isolated checks can immediately expose irrespective of whether a selected bit of logic is Doing the job as envisioned. Every time a take a look at fails, you quickly know the place to search, substantially lowering the time spent debugging. Device checks are In particular valuable for catching regression bugs—concerns that reappear right after previously remaining fastened.
Following, integrate integration checks and conclusion-to-conclude exams into your workflow. These aid make sure that various portions of your application work alongside one another efficiently. They’re especially useful for catching bugs that come about in sophisticated systems with various factors or providers interacting. If some thing breaks, your assessments can let you know which Element of the pipeline failed and under what ailments.
Creating checks also forces you to Imagine critically about your code. To test a element effectively, you would like to grasp its inputs, expected outputs, and edge situations. This level of knowledge Normally sales opportunities to better code construction and much less bugs.
When debugging a concern, writing a failing examination that reproduces the bug can be a strong starting point. Once the examination fails continuously, you'll be able to center on fixing the bug and observe your exam move when The difficulty is resolved. This strategy makes certain that the same bug doesn’t return Later on.
Briefly, crafting tests turns debugging from a discouraging guessing activity into a structured and predictable method—helping you catch a lot more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult situation, it’s uncomplicated to be immersed in the problem—looking at your display for hrs, striving solution following Remedy. But The most underrated debugging instruments is solely stepping absent. Having breaks allows you reset your mind, reduce aggravation, and often see the issue from a new perspective.
When you are also close to the code for also extended, cognitive tiredness sets in. You could commence overlooking apparent mistakes or misreading code that you simply wrote just hours earlier. In this point out, your Mind becomes fewer economical at trouble-fixing. A short walk, a espresso crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of builders report obtaining the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly during for a longer period debugging periods. Sitting in front of a display screen, mentally caught, is not only unproductive but will also draining. Stepping away allows you to return with renewed Electrical power plus a clearer state of mind. You may perhaps quickly recognize a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you prior to.
In the event you’re trapped, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 minute crack. Use that time to maneuver about, extend, or do some thing unrelated to code. It may come to feel counterintuitive, Particularly underneath tight deadlines, nonetheless it actually brings about faster and simpler debugging in the long run.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart system. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of solving it.
Understand From Each Bug
Each and every bug you come upon is more than just A brief setback—It is really an opportunity to expand being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you something useful in case you make the effort to reflect and examine what went Mistaken.
Start out by inquiring yourself a few crucial queries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are caught before with improved tactics like device tests, code assessments, or logging? The answers frequently reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding routines moving ahead.
Documenting bugs will also be a wonderful pattern. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or widespread problems—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug along with your peers is often Specially effective. Whether or not it’s via a Slack information, a short create-up, or A fast expertise-sharing session, aiding others steer clear of the identical issue boosts workforce effectiveness and cultivates a stronger Mastering tradition.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as essential portions of your advancement journey. After all, several of the best builders are not those who write best code, but those who repeatedly learn from their problems.
In the end, Every read more single bug you fix adds a different layer for your ability established. So upcoming time you squash a bug, have a second to mirror—you’ll occur away a smarter, far more able developer due to it.
Conclusion
Improving upon your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It makes you a more successful, self-assured, and capable developer. The following time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be better at Everything you do.